There have been some stellar improvements to SureCart recently, and one of those is that now included in the payload are all the details you need to begin to automate shipping. The quantities and weights are now passed within the payload correctly, and finally give you enough details to begin looking at automation.
While I haven’t currently got a use case for a physical SureCart store, I did want to look at how this could be automated and the data feed into my automator of choice, FlowMattic, and this is what I was able to achieve quite simply and easily.
Now Flowmattic is capable of doing this with its own native integration, but I’d need to filter the details, so I decided to build a small script to grab the details on checkout confirmed and add an action hook for flowmattic to listen to.
// 1) Hook into SureCart checkout confirmed
add_action( 'surecart/checkout_confirmed', 'sc_send_flowmattic_payload', 10, 1 );
function sc_send_flowmattic_payload( \SureCart\Models\Checkout $checkout ) {
// 2) Extract customer
$customer_name = trim( $checkout->first_name . ' ' . $checkout->last_name );
$customer_email = $checkout->email;
$customer_phone = $checkout->phone;
// 3) Extract shipping address (may be null)
$sa = $checkout->shipping_address ?: new stdClass();
// 4) Build items loop
$items = [];
foreach ( $checkout->line_items->data as $item ) {
$unfulfilled = $item->quantity - $item->fulfilled_quantity;
$weight_unit = $item->price->product->weight_unit ?? '';
$items[] = [
'title' => $item->price->product->name,
'options' => $item->variant_option_names ?: [],
'sku' => $item->price->product->sku ?? '',
'unfulfilled_qty' => $unfulfilled,
'weight' => $item->unit_g_weight,
'weight_unit' => $weight_unit,
'amount' => $item->total_amount,
];
}
// 5) Order-level data
$shipping_method = $checkout->selected_shipping_choice;
$shipping_amount = $checkout->shipping_amount;
$order_total_amount = $checkout->total_amount;
$order_currency = $checkout->currency;
// 6) Assemble payload, include custom_id for Flowmattic metadata
$payload = [
'custom_id' => $checkout->id, // unique identifier
'name' => $customer_name,
'email' => $customer_email,
'phone' => $customer_phone,
'street_line_1' => $sa->line_1 ?? '',
'street_line_2' => $sa->line_2 ?? '',
'city' => $sa->city ?? '',
'state' => $sa->state ?? '',
'postal_code' => $sa->postal_code ?? '',
'country' => $sa->country ?? '',
'items' => $items,
'shipping_method' => $shipping_method,
'shipping_amount' => $shipping_amount,
'total_amount' => $order_total_amount,
'currency' => $order_currency,
];
// 7) Fire a custom action for Flowmattic to catch
do_action( 'flowmattic_surecart_checkout_confirmed', $payload );
}
PHP
Using this script, we get a nice product list and receive data in FlowMattic as shown below
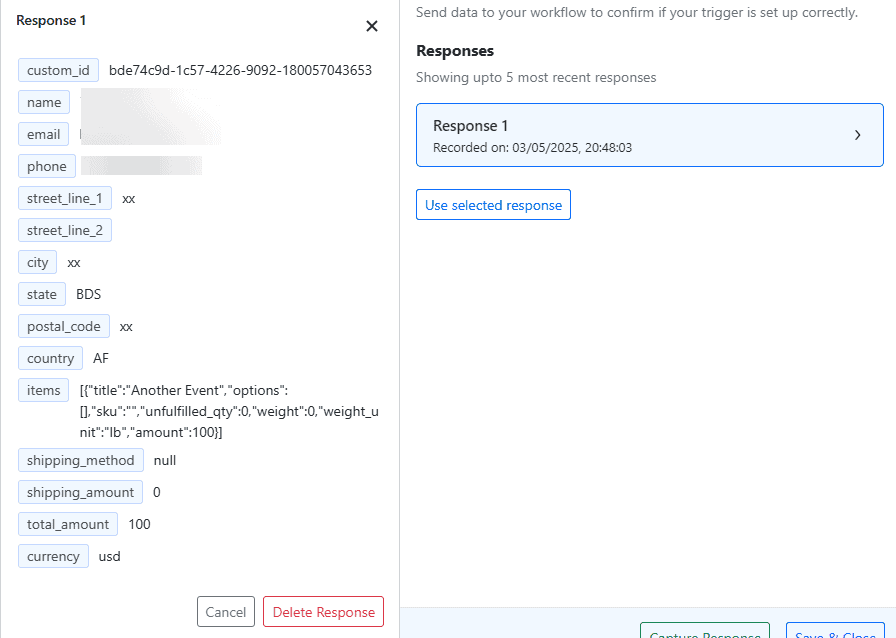
With the outgoing API module, it is now totally possible to generate shipping labels for sales made in SureCart using FlowMattic