A question was asked on the SureCrafted Facebook group about smart tag and the lack of filters within the documentation, specifically about using an ACF option page field (email) as the email to send email notifications to on submissions of a SureForms.
I have spent a fair amount of time within the code of SureForms and as such knew there where filters to apply to do this specifically the following two: “srfm_smart_tag_list” & “srfm_parse_smart_tags”
Both are located in inc\smart-tags.php
/**
* Smart Tag List.
*
* @since 0.0.1
* @return array<mixed>
*/
public static function smart_tag_list() {
return apply_filters(
'srfm_smart_tag_list',
[
'https://cms.techarticles.co.uk' => __( 'Site URL', 'sureforms' ),
'{admin_email}' => __( 'Admin Email', 'sureforms' ),
'Tech Articles' => __( 'Site Title', 'sureforms' ),
'{form_title}' => __( 'Form Title', 'sureforms' ),
'{ip}' => __( 'IP Address', 'sureforms' ),
'{http_referer}' => __( 'HTTP Referer URL', 'sureforms' ),
'{date_mdy}' => __( 'Date (mm/dd/yyyy)', 'sureforms' ),
'{date_dmy}' => __( 'Date (dd/mm/yyyy)', 'sureforms' ),
'{user_id}' => __( 'User ID', 'sureforms' ),
'{user_display_name}' => __( 'User Display Name', 'sureforms' ),
'{user_first_name}' => __( 'User First Name', 'sureforms' ),
'{user_last_name}' => __( 'User Last Name', 'sureforms' ),
'{user_email}' => __( 'User Email', 'sureforms' ),
'{user_login}' => __( 'User Username', 'sureforms' ),
'{browser_name}' => __( 'Browser Name', 'sureforms' ),
'{browser_platform}' => __( 'Browser Platform', 'sureforms' ),
'{embed_post_id}' => __( 'Embedded Post/Page ID', 'sureforms' ),
'{embed_post_title}' => __( 'Embedded Post/Page Title', 'sureforms' ),
'{get_input:param}' => __( 'Populate by GET Param', 'sureforms' ),
'{get_cookie:cookie_name}' => __( 'Cookie Value', 'sureforms' ),
]
);
}
PHP
/**
* Smart Tag Callback.
*
* @param string $tag smart tag.
* @param array<mixed>|null $submission_data data from submission.
* @param array<mixed>|null $form_data data from form.
* @since 0.0.1
* @return mixed
*/
public static function smart_tags_callback( $tag, $submission_data = null, $form_data = null ) {
$parsed_tag = apply_filters( 'srfm_parse_smart_tags', null, compact( 'tag', 'submission_data', 'form_data' ) );
if ( ! is_null( $parsed_tag ) ) {
return $parsed_tag;
}
switch ( $tag ) {
case 'https://cms.techarticles.co.uk':
return site_url();
case '{admin_email}':
return get_option( 'admin_email' );
case 'Tech Articles':
return get_option( 'blogname' );
case '{form_title}':
if ( ! empty( $form_data ) && is_array( $form_data ) && ! empty( $form_data['form-id'] ) ) {
$id = absint( $form_data['form-id'] );
$post = get_post( $id );
if ( $post instanceof \WP_Post ) {
return esc_html( $post->post_title ) ?? '';
}
}
return '';
case '{http_referer}':
return wp_get_referer();
case '{ip}':
return self::get_the_user_ip();
case '{date_dmy}':
case '{date_mdy}':
return self::parse_date( $tag );
case '{user_id}':
case '{user_display_name}':
case '{user_first_name}':
case '{user_last_name}':
case '{user_email}':
case '{user_login}':
return self::parse_user_props( $tag );
case '{browser_name}':
case '{browser_platform}':
return self::parse_browser_props( $tag );
case '{embed_post_id}':
case '{embed_post_title}':
case '{embed_post_url}':
return self::parse_post_props( $tag );
default:
if ( strpos( $tag, 'get_input:' ) || strpos( $tag, 'get_cookie:' ) ) {
return self::parse_request_param( $tag );
}
if ( 0 === strpos( $tag, '{form:' ) ) {
return self::parse_form_input( $tag, $submission_data, $form_data );
}
break;
}
}
PHP
So with this in mind I created the following snippet to add the {options_email} smart tag which is expandable for other option page items and other variables you may need to turn into a smart tag for use within SureForms
<?php
add_filter('srfm_smart_tag_list', 'add_options_email_to_smart_tag_list');
function add_options_email_to_smart_tag_list($tags) {
$tags['{options_email}'] = __('Options Email (ACF)', 'sureforms');
return $tags;
}
add_filter('srfm_parse_smart_tags', 'replace_options_email_tag', 10, 3);
function replace_options_email_tag($parsed_tag, $args) {
// Check if the tag is {options_email}
if ($args['tag'] === '{options_email}') {
// Get the email address from the ACF options page
$email_address = get_field('email_address', 'option'); // Replace with your ACTUAL option page field!
if ($email_address) {
return $email_address; // Return the email address for replacement
} else {
error_log('ACF email address not found for {options_email}');
return ''; // Return empty string if not found
}
}
return $parsed_tag; // Return the original tag if not {options_email}
}
PHP
You must replace the get_field in line 13 with your actual option name
This then allows you to add the following tag {options_email} into your sent email to field as per the below, the first filter adds optons_email and the second replaces it with what is set within your option page
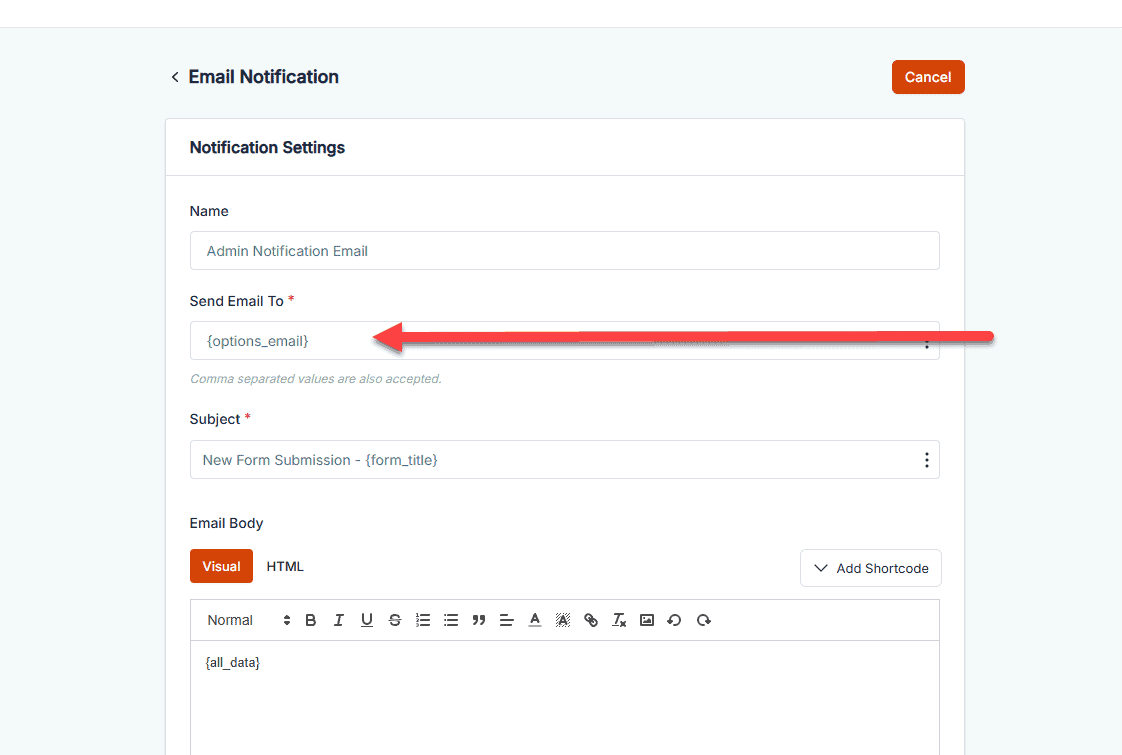
This snippet and method could be used to add all sorts of additional useable smart tags including unique data from the website and other option page options, this could be useful too if you want to add more than one email to notifications add them to the CC field from the options page and expand the available email addresses you can send too, and easy to change on an unlimited number of forms in one location.
You can view a video of the implementation here: